LinkedIn Professional - Using LinkedIn's API
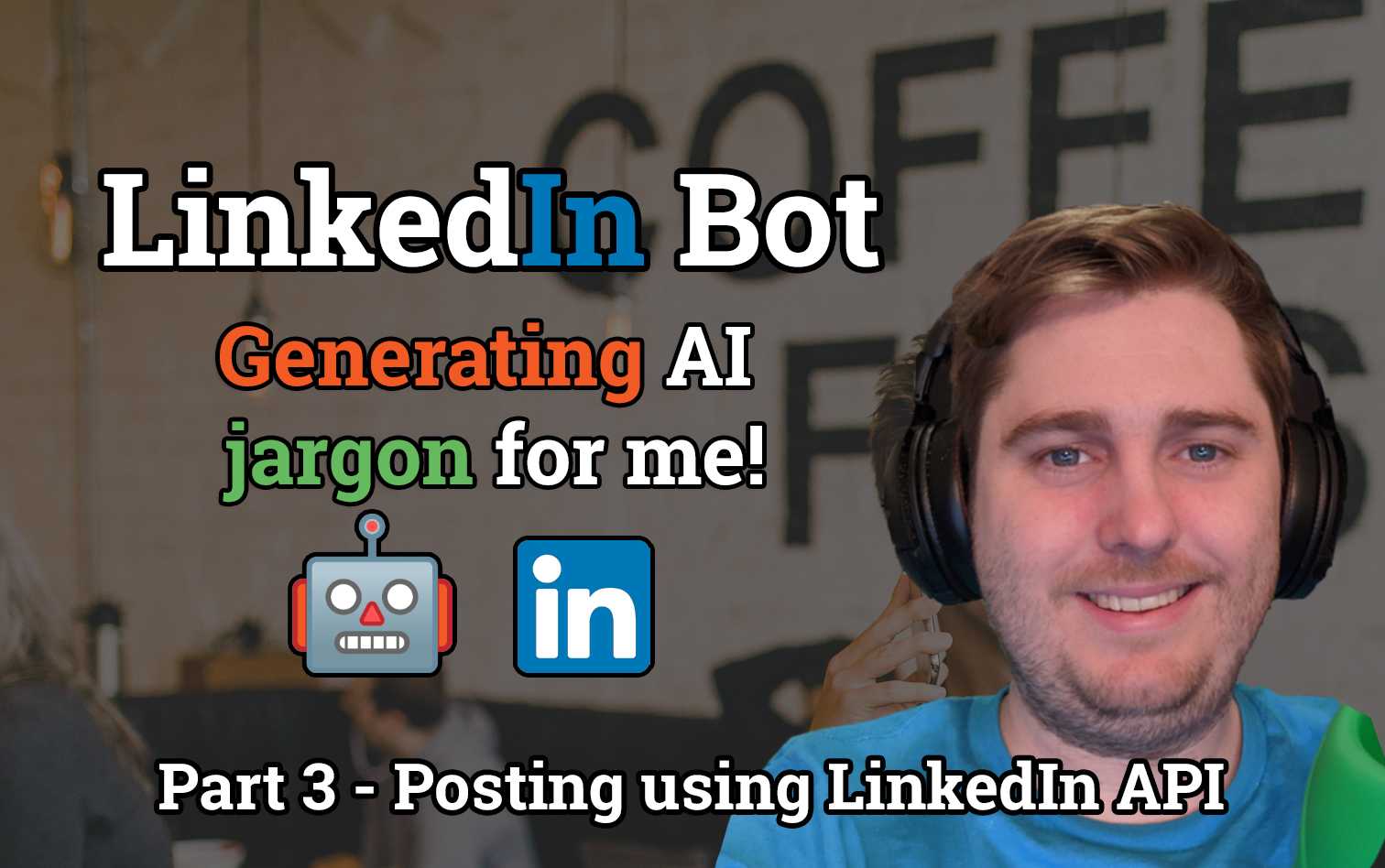
Table of Contents
- LinkedIn Professional - Project Overview
- LinkedIn Professional - Generating Tech Jargon with DeepAI Text Generator
- LinkedIn Professional - Using LinkedIn's API
- LinkedIn Professional - Deploying a Serverless project
LinkedIn Professional - Using LinkedIn's API
Now that we have our technical jargon generated it is ready to be shared on LinkedIn with your peers. Similarly to other social networks post Cambridge Analytica; it is not as simple to interact with the platform.
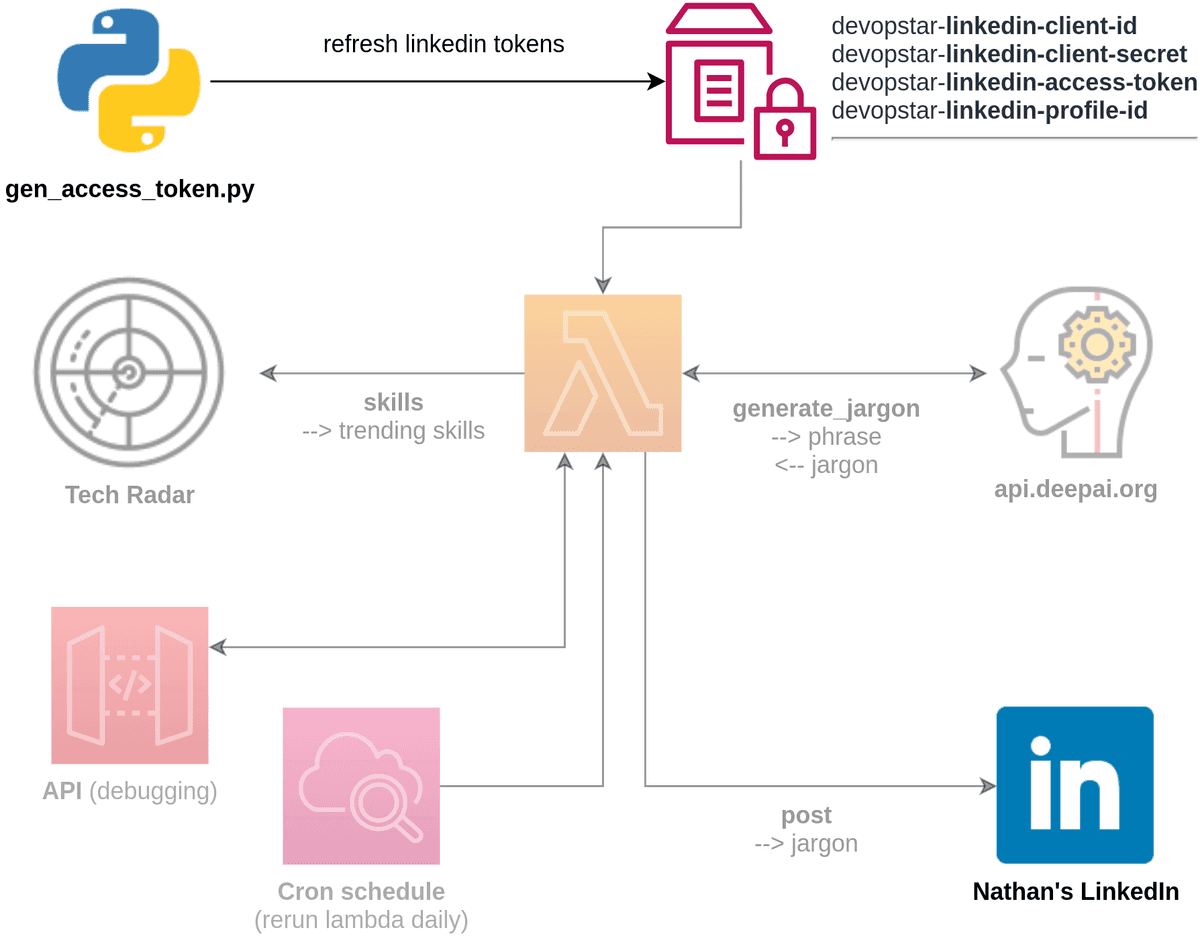
Prerequisites
To deploy this project yourself you will require a couple things to be pre-setup:
- AWS CLI (with credentials)
- LinkedIn Account (person) and a LinkedIn 'Company' - You will need a LinkedIn Company to exist under your standard LinkedIn Account, this can be done from the LinkedIn company setup page. For the purpose of this guide I created one for DevOpStar.
Creating a LinkedIn Developer App
With the company create, now navigate to the LinkedIn Developers page and click Create app.
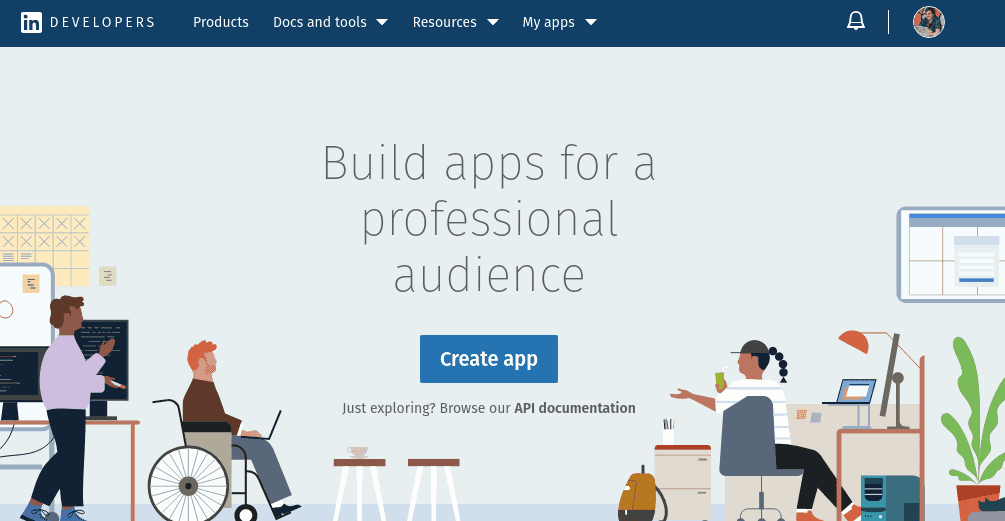
Go through and punch in whatever details you want for the app description. I called my app Technical Professional Excellence #1 which proves that LinkedIn don't have any real vetting process (which is great for us).
Be sure to tie your previously created LinkedIn Page to your app as well, it is required so you won't be able to get past this step without it.
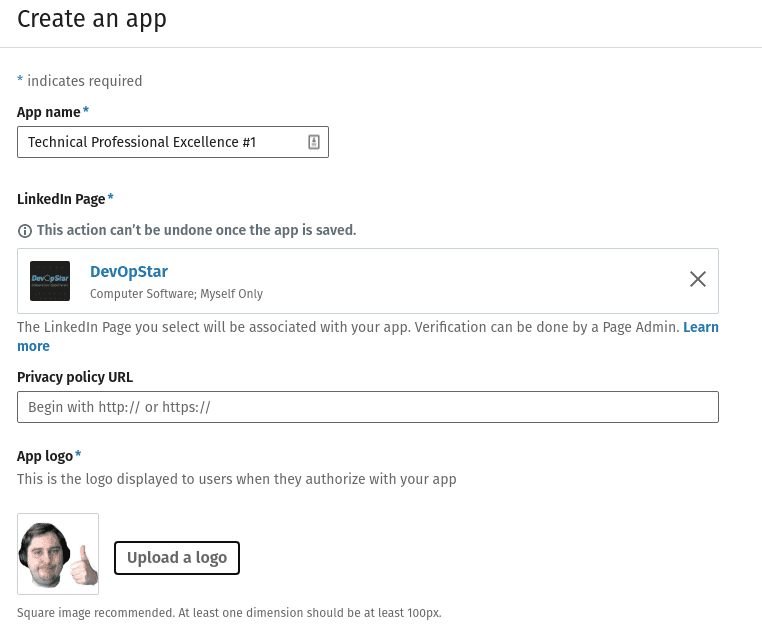
Once your app is created just on over to the Auth
tab and retrieve your Client ID and Client Secret. Store these somewhere safe for when they are needed later on.
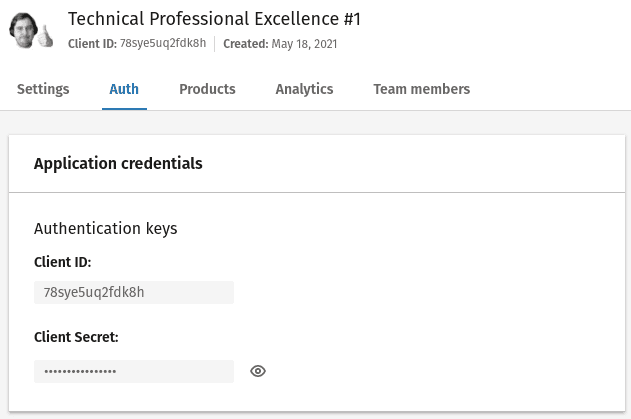
To make life easier for you later, I would recommend storing these two variables in AWS Parameter store (as I have done in the linkedin-professional repository).
To do this run the following commands substituting in the Client ID and Client Secret.
aws ssm put-parameter --overwrite \
--name devopstar-linkedin-client-id \
--type String \
--value $CLIENT_ID_GOES_HERE
aws ssm put-parameter --overwrite \
--name devopstar-linkedin-client-secret \
--type String \
--value $CLIENT_SECRET_GOES_HERE
The final step that has to be completed is to enable product support for the app. This is done under the Products
tab and you will need to select the following two products to to be setup
- Sign In with LinkedIn - Allows the app to act as a given user that signs in with it. This just means that the app will be able to pull information about us (the user that needs to post to LinkedIn).
- Share on LinkedIn - Allows the app to share posts on LinkedIn.
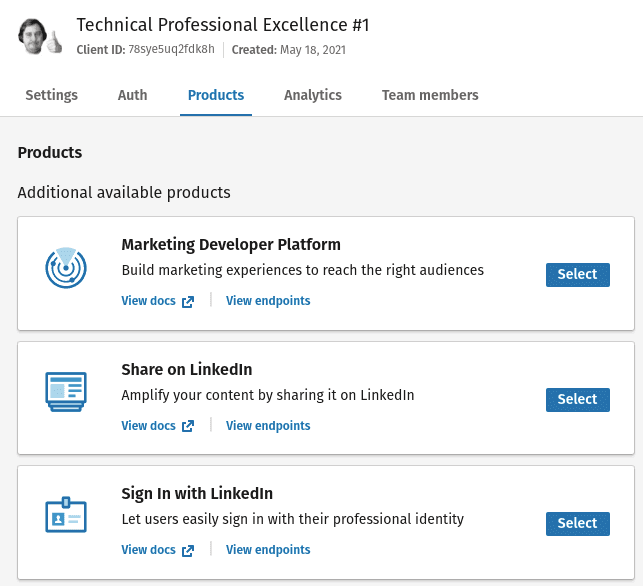
Authenticating with a LinkedIn App
Now that the app is setup it is time to test we can authenticate with it, and generate an access token for our user that can be used to post.
This process is done via a fairly complicated 2 step auth process that I've automated in the linkedin-professional/gen_access_token.py file
To first generate a LinkedIn Client Auth Code you should run the create_user_code() block which will create an authorization request on behalf of your personal LinkedIn user to the LinkedIn App we created.
def create_user_code():
# Generate a random string to protect against cross-site request forgery
letters = string.ascii_lowercase
CSRF_TOKEN = ''.join(random.choice(letters) for i in range(24))
# Request authentication URL
auth_params = {'response_type': 'code',
'client_id': CLIENT_ID,
'redirect_uri': REDIRECT_URI,
'state': CSRF_TOKEN,
'scope': 'r_liteprofile,r_emailaddress,w_member_social'}
html = requests.get(
"https://www.linkedin.com/oauth/v2/authorization", params=auth_params)
# Print the link to the approval page
print(html.url)
A HTML URL will be printed out when you run the python code above that when navigated to will prompt you to authenticate with the LinkedIn app.
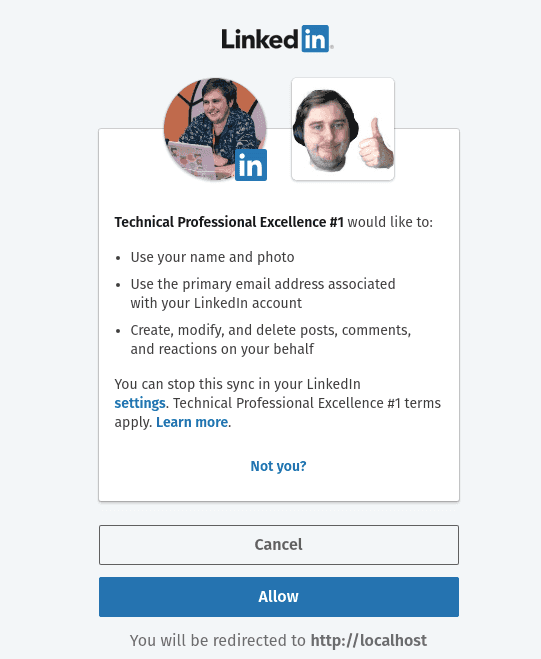
Click Allow and the page should redirect and say "This site can't be reached", this is expected! Note that a URL should have changed in the URL bar of your browser that will look something like this:
http://localhost:8000/?code=AQRqIJqepo61rTNZYeQVqIOcGTW1KfWAuHuwepcwLNQ5ZD4OyEDIhACaLKSHjfJKlhasYI2yJMUOT-rJJz2f4lTNcqtRPtMg1asdlkfjslkadjfjcmjMN-tPxb148Oe5kX-6CbqQ2PhWdvBdC3ma5nGeO4hKnieN9Yv3aU1kqq5waY1C6DOj_WE5mrOFWnUSQDvTXwaijvIrEB5pFvIGL1pA
The long string under the code
URL paramter is the client auth code that we will need to use to generate an access token with the permissions needed to post from our user.
Again, to make your life easier I recommend adding this paramter to a AWS Paramter store object by running the following command
aws ssm put-parameter --overwrite \
--name devopstar-linkedin-auth-code \
--type String \
--value $CLIENT_AUTH_CODE
Generating A LinkedIn Access Token
The final auth step that we need to take is to generate an access token capable of performing actions as a given user from our LinkedIn app.
I have automated much of this process in the function get_access_token() which can be seen below:
def get_access_token():
AUTH_CODE = client.get_parameter(
Name='devopstar-linkedin-auth-code')['Parameter']['Value']
CLIENT_SECRET = client.get_parameter(
Name='devopstar-linkedin-client-secret')['Parameter']['Value']
ACCESS_TOKEN_URL = 'https://www.linkedin.com/oauth/v2/accessToken'
qd = {'grant_type': 'authorization_code',
'code': AUTH_CODE,
'redirect_uri': REDIRECT_URI,
'client_id': CLIENT_ID,
'client_secret': CLIENT_SECRET}
response = requests.post(ACCESS_TOKEN_URL, data=qd, timeout=60)
response = response.json()
access_token = response['access_token']
print("Access Token:", access_token)
print("Expires in (seconds):", response['expires_in'])
client.put_parameter(
Name='devopstar-linkedin-access-token',
Type='String',
Value=access_token,
Overwrite=True
)
This function pulls together all the auth details we have been defining so far and will create us a LinkedIn access token that will be valid for 60 days capable of posting as our LinkedIn user.
There is also another function in this file called get_linkedin_profile_id() that will retrieve our users LinkedIn ID and stores it for us in AWS Paramter store.
To run both of these functions automatically, uncomment them AND recomment create_user_code()
so it doesn't run again.
if __name__ == "__main__":
# Run me first
#create_user_code()
# Run me second
get_access_token()
# Run me to test
get_linkedin_profile_id()
LinkedIn Authentication Summary
Congratulations! You have finished up the section of setting up LinkedIn authentication for a user via a LinkedIn App.
In the final section we will wire everything we have setup so far together and deploy it to AWS to run on AWS Lambda.